In this tutorial, I’ll be converting a text string to an audio file using PHP and an API endpoint provided by the ElevenLabs text-to-speech service.
As a PHP developer, you can use these examples in various applications like accessibility widgets, e-learning platforms, etc.
Why Use the ElevenLabs API
The ElevenLabs API has some advantages over competing services like Azure Text to Speech, Text-to-Speech AI, and Amazon Polly:
- It doesn’t require a complex cloud service configuration. You just need to get your API Key from your account and paste it into your application.
- It has plans with a fixed number of characters, and you can avoid the risks associated with pay-as-you-go services.
- It has voice cloning capabilities. Right now, this feature is not available in the major competitors mentioned above.
Implement Your Text-to-Speech Converter
Get the API key
As with most API services, you need an API key to authenticate your requests.
The API key is available on the ElevenLabs site. Specifically, you can get it with this procedure:
- Log in to your ElevenLabs account.
- Click the user icon at the top-right section of the screen.
- Select the Profile + API Key menu item.
- Copy the API Key value in a local file.
You now have the API Key; in the next section, this value will be used in the PHP implementation of the text-to-speech converter.
Configure the ElevenLabs Request Options
To begin, we must prepare a few variables to authenticate and configure our request.
The first variable we must set up is the one that stores the ElevenLabs API key to authenticate the request.
// Note: Replace the asterisks with the API key available in your ElevenLabs user profile $elevenlabs_api_key = "********************************"
Then, set the text that should converted to audio:
// Text to convert $text = "Speak this";
Now, set the Voice ID. Note that you can get a list of the available voices with the related Voice ID by performing a request to a dedicated API endpoint. For more details, this is a voice fetch example provided by ElevenLabs.
The code below includes the Voice ID that allows us to select a female voice named “Rachel”.
// Voice ID (find available voices using the API) $voiceId = '21m00Tcm4TlvDq8ikWAM';
Now, configure the API endpoint URL. This is where our script will send the requests.
// API endpoint URL $url = "https://api.elevenlabs.io/v1/text-to-speech/" . $voiceId;
Select the model. ElevenLabs currently has multiple speech synthesis models. Our example will use Eleven Multilingual v2, the latest multi-language model, with support for 29 languages.
$model_id = 'eleven_multilingual_v2';
We have now stored in PHP variables all the parameters needed to perform a basic request to the ElevenLabs API. In the next section, we will see how to perform the actual request.
Perform the HTTP Request
The cURL Request Template Provided by ElevenLabs
ElevenLabs provides an online form that allows us to generate a ready-to-use PHP script that uses cURL to perform an API request. To use this tool:
- Proceed to this page and insert basic parameters like your API key, the voice ID you want to use, and the text that should be converted to audio.
- Click the “Send” button.
- If the request is successful, copy the generated implementation code for PHP.
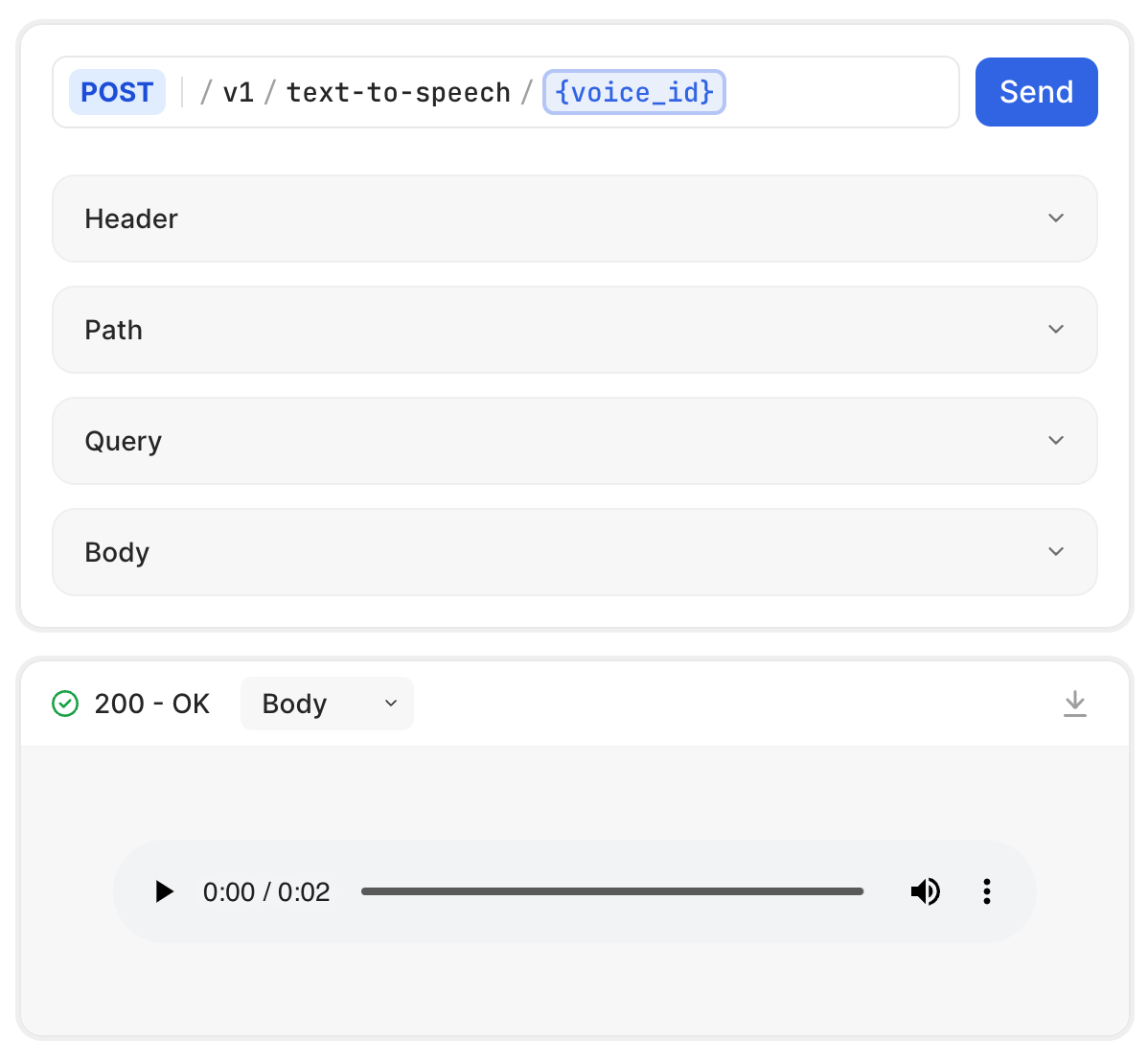
The following code provided by ElevenLabs will be used as a starting point in our implementation.
<?php $curl = curl_init(); curl_setopt_array($curl, [ CURLOPT_URL => "https://api.elevenlabs.io/v1/text-to-speech/21m00Tcm4TlvDq8ikWAM", CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "POST", CURLOPT_POSTFIELDS => "{\n \"model_id\": \"eleven_multilingual_v1\",\n \"text\": \"a\"\n}", CURLOPT_HTTPHEADER => [ "Content-Type: application/json", "xi-api-key: ********************************" ], ]); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { echo $response; }
Our Implementation
First, define the request headers. Here, the xi-api-key
header is used to pass the API Key.
// Set up the request headers $headers = [ "Content-Type: application/json", "xi-api-key: $elevenlabs_api_key" ];
Other ElevenLabs parameters should be added in the request body:
// Set up the request parameters, if any $data = json_encode( array( 'text' => $text, 'model_id' => $model_id ) );
Note that additional configuration options can be used in the request. Specifically, you can use these additional options by adding them to the request body. For example, here I configured some additional parameters, specifically the stability, similarity boost, style, and use_speaker_boost:
// Set up the request parameters, if any $data = json_encode( array( 'text' => $text, 'voiceId' => $voiceId, 'model_id' => $model_id, 'voice_settings' => array( 'stability' => 0, 'similarity_boost' => 0, 'style' => 0, 'use_speaker_boost' => 1 ) ) );
You can now submit the request with cURL (this part is an augmented version of the code generated by the ElevenLabs tool described above):
$curl = curl_init(); curl_setopt_array( $curl, [ CURLOPT_URL => $url, CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "POST", CURLOPT_POSTFIELDS => $data, CURLOPT_HTTPHEADER => $headers, ] ); $response = curl_exec( $curl ); $err = curl_error( $curl ); curl_close( $curl );
Store the Audio Stream in a File
We can now handle the response stored in $response
variable. There are two cases to cover. More precisely, if the request fails, we display the error on the HTML page. If the request is successful, we create an mp3 file using file_put_content()
with the bytes in the response.
if ( $err ) { echo "cURL Error #:" . $err; } else { // A valid response includes the audio stream. $audio_stream = $response; // Set the location where the mp3 file will be stored. $file_path = '/home/yoursite/public_html/mp3/example.mp3'; // Save the audio data as an MP3 file on the server. $res = file_put_contents( $file_path, $audio_stream ); // Print a success message. echo "MP3 file successfully saved."; }
Alternative Methods to Perform HTTP Requests With PHP
Based on the PHP environment and your preferences, you can use different functions to perform the HTTP requests used to communicate with the ElevenLabs API.
These are alternative methods to perform HTTP requests with PHP:
- PHP cURL without a framework. (the method used in this tutorial)
- The Guzzle PHP HTTP client.
- If you are developing the text-to-speech converter for a WordPress plugin or theme, I recommend using
wp_remote_request()
and the relatedwp_remote_retrieve_body()
to retrieve the response body.
Note: To properly perform the HTTP request, you have to enable the cURL PHP extension or use Guzzle with a custom HTTP transport. Failing to do so will result in PHP errors.
A WordPress Plugin to Convert Text to Speech With ElevenLabs
If you are working with WordPress, our plugin Real Voice includes an integration for ElevenLabs. In that case, to perform a text-to-speech conversion, you have only to include the ElevenLabs API key in the provided option field.
Note
API services constantly change, and I can’t guarantee that the specific PHP implementation presented in the article will still be relevant when you read this article. Please always get the latest implementation details in the ElevenLabs official documentation.