Select2 is a JavaScript library used to create an enhanced replacement of the select
element displayed by the browser.
Recently we used this library multiple times to create well-styled and searchable select boxes in our WordPress plugins, so I decided to write an article on this subject.
Install Select2 in WordPress
In a WordPress plugin or a custom theme, you can use the admin_enqueue_scripts
action hook to add the resources required to enable Select2.
Please note that I loaded the resources from a CDN in the example below, but you can use local resources by downloading all the files from the Select 2 GitHub repository.
function admin_enqueue_scripts_callback(){ //Add the Select2 CSS file wp_enqueue_style( 'select2-css', 'https://cdn.jsdelivr.net/npm/select2@4.1.0-rc.0/dist/css/select2.min.css', array(), '4.1.0-rc.0'); //Add the Select2 JavaScript file wp_enqueue_script( 'select2-js', 'https://cdn.jsdelivr.net/npm/select2@4.1.0-rc.0/dist/js/select2.min.js', 'jquery', '4.1.0-rc.0'); //Add a JavaScript file to initialize the Select2 elements wp_enqueue_script( 'select2-init', '/wp-content/plugins/select-2-tutorial/select2-init.js', 'jquery', '4.1.0-rc.0'); } add_action( 'admin_enqueue_scripts', 'admin_enqueue_scripts_callback' );
You can now add the HTML of the select element in an administrative menu. If you don’t know how to create a menu, check out the Top-Level Menus documentation page on WordPress.org.
<select id="example-select"> <option>Option 1</option> <option>Option 2</option> <option>Option 3</option> </select>
For a select multiple simply add the multiple attribute:
<select id="example-select" multiple> <option>Option 1</option> <option>Option 2</option> <option>Option 3</option> </select>
The initialization of the Select2 control performed in the select2-init.js initialization file:
jQuery(document).ready(function($) { $('#example-select').select2(); });
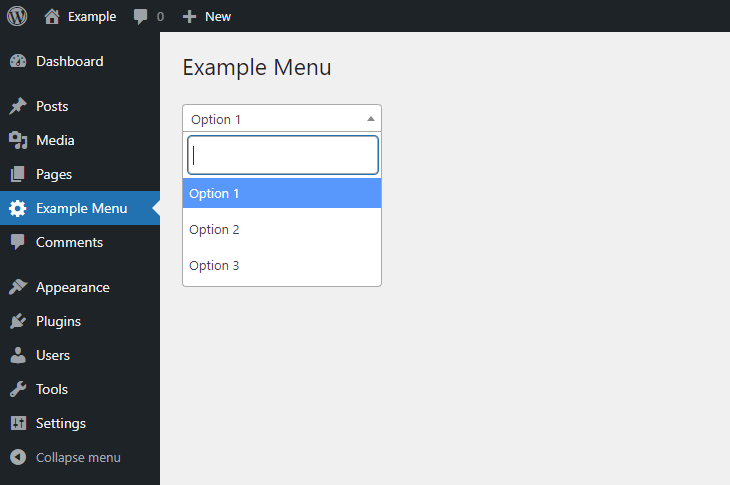
Configure the options
Select2 comes with more than 30 options. You can use these options by passing a JavaScript object with the options values during the plugin initialization. In this example, I configured one single option used to control the width of the select control.
$('#example-select').select2({ width: '200px', });
An alternative method is to add the options as data attributes of the select
element.
<select id="example-select" data-width="200px"> <option>Option 1</option> <option>Option 2</option> <option>Option 3</option> </select>
For more information, here you can find a complete list of the select2 option.
Useful Select2 features for WordPress developers
Grouping
When the listed options belong to different categories, you can create groups of options. To do this, wrap the groups in the optgroup
tag as you usually do when Select2 is not used.
<select id="example-select"> <optgroup label="First group"> <option>Option 1</option> <option>Option 2</option> <option>Option 3</option> </optgroup> <optgroup label="Second group"> <option>Option 4</option> <option>Option 5</option> </optgroup> </select>
Select options
To programmatically select an item for a Select2 control, use the jQuery .val()
method.
This example demonstrates how to select a specific option of the select2 control with a click on a button:
$(document.body).on('click', '#select-option' , function(){ $('#example-select').val('2'); $('#example-select').trigger('change'); });
Retrieve the value of the element
You can return an array of objects with the data of the current selection by providing the string ‘data’ to the select2 method.
In this example, the data of a select element are displayed with an alert message after clicking on a button.
$(document.body).on('click', '#select-option' , function(){ //Store the data of the select2 element in a variable let dataObject = $('#example-select').select2('data');; //An alert message with the id of the selected element is generated alert(dataObject[0]['id']); });
Internationalization
If your theme or plugin that uses Select2 is distributed in languages different than English, or if you want to customize the text strings displayed in the Select control, you can use the internationalization feature.
The first method is to set the code of the language in the language property during the initialization. In this example, the “it” code indicates the Italian language.
$('#example-select').select2({ language: "it" });
The second method is to provide custom translation string to all the translatable elements:
$('#example-select').select2({ language: { errorLoading: function() {return 'I risultati non possono essere caricati.';}, inputTooLong: function(e) { var n = e.input.length - e.maximum, t = 'Per favore cancella ' + n + ' caratter'; return t += 1 !== n ? 'i' : 'e'; }, inputTooShort: function(e) { return 'Per favore inserisci ' + (e.minimum - e.input.length) + ' o più caratteri'; }, loadingMore: function() {return 'Caricando più risultati…';}, maximumSelected: function(e) { var n = 'Puoi selezionare solo ' + e.maximum + ' element'; return 1 !== e.maximum ? n += 'i' : n += 'o', n; }, noResults: function() {return 'Nessun risultato trovato';}, searching: function() {return 'Sto cercando…';}, removeAllItems: function() {return 'Rimuovi tutti gli oggetti';}, } });
Style customizations
When the style of Select2 control is important, add a stylesheet to the WordPress back-end.
//Add the Select2 CSS file wp_enqueue_style( 'select2-custom-css', '/wp-content/plugins/select-2-tutorial/select2-custom.css', array());
Then use this file to apply custom CSS rules. You can find the selectors by inspecting the Select2 control with the Chrome DevTools.
#example-select{ border-color: red; } #example-select option{ font-weight: bold; }
Alternatively you can change theme:
$('#example-select').select2({ theme: 'classic' });
Advanced options
Other common needs of WordPress plugin developers are covered by the features included in Select2. Some notable examples are listed below.
Templating
You can customize the results of the Select2 control with the templating feature. For example, you can generate images and text with a custom layout for each option.
Automatic tokenization
With the automatic tokenization of Select2, you can create new options by adding words in the search field.
Customize the search results
You can use a custom function to filter search results with the matcher property of the configuration object.
function customSearch(params, data) { /** * Here use the submitted string and the value of the options to decide * which results should be returned. */ } $('#example-select').select2({ matcher: customSearch });
AJAX requests with WordPress
With this feature, you can search in remote data, such as the JSON data of a REST API. For more information, visit the AJAX page.
Alternatives to Select2 for WordPress developers
The main alternative to Select2 is the Chosen library, which still works for the most part, but it’s no longer support. In case you are interested in the Chosen library, read this article.
If you are developing Gutenberg blocks, you might also consider ReactSelect, an enhanced select control for ReactJS.