This article lists different methods to implement a random order on a WordPress website.
Apply a random order in WordPress with a custom solution
Display random posts on the homepage
In the script below, I use pre_get_posts
to modify the WordPress query. In addition, I adopt the is_home()
and is_main_query()
conditional tags to limit the application of the random order exclusively on the main query of the homepage.
function posts_random_order( $query ) { if ( $query->is_main_query() && $query->is_home() ) { $query->set( 'orderby', 'rand' ); } } add_action( 'pre_get_posts', 'posts_random_order' );
Display the posts of an archive page in random order
The following example uses WP_Query
with the parameter orderby
to randomize the archive page of a custom post type. You should place this code in your theme template file in place of the default loop.
$query = new WP_Query( array( 'post_type' => 'movie', 'orderby' => 'rand', 'posts_per_page' => '-1' ) ); if ( $query->have_posts() ): while ( $query->have_posts() ) : $query->the_post(); //Echo the title, the excerpt, etc. endwhile; endif;
Display a list of posts in random order
This other solution creates a shortcode that displays a list of posts in random order. This new shortcode will support four parameters used to define the resulting content.
Note that this implementation is similar to the one used in the previous section. In this case, however, the loop is wrapped in a shortcode callback.
function display_random_posts( $atts ) { $post_type = sanitize_key( $atts['post_type'] ); $posts_per_page = intval( $atts['posts_per_page'], 10 ); $args = array( 'post_type' => $post_type, 'posts_per_page' => $posts_per_page, 'post_status' => 'publish', 'orderby' => 'rand', ); $query = new WP_Query( $args ); // Display the list of posts if ( $query->have_posts() ) { while ( $query->have_posts() ) { $query->the_post(); //Echo the related post thumbnail, title, etc. echo '<p>' . esc_html( get_the_title() ) . '</p>'; } } wp_reset_query(); } add_shortcode( 'random_posts', 'display_random_posts' ); add_filter( 'widget_text', 'do_shortcode' );
To use this new shortcode in your WordPress site, add this code in a post:
[random_posts post_type="post" posts_per_page="3"]
Note that the last line of the script enables using the shortcode in a widget area. With this instruction present in your shortcode implementation, you can, for example, create custom lists of posts in random order in the site sidebar.
Random order in WordPress without coding
In this section, I will share ideas to display WordPress content in random order without using code.
Change the post date manually from the post editor
A simple method to generate an archive page in random order is to change the date of all the articles.
You can do this manually from the administrative side of WordPress. Specifically, start by visiting the Posts -> All Posts menu. Then edit one article. While editing the article, use the Date field to set a random date. Repeat the process for all your articles.
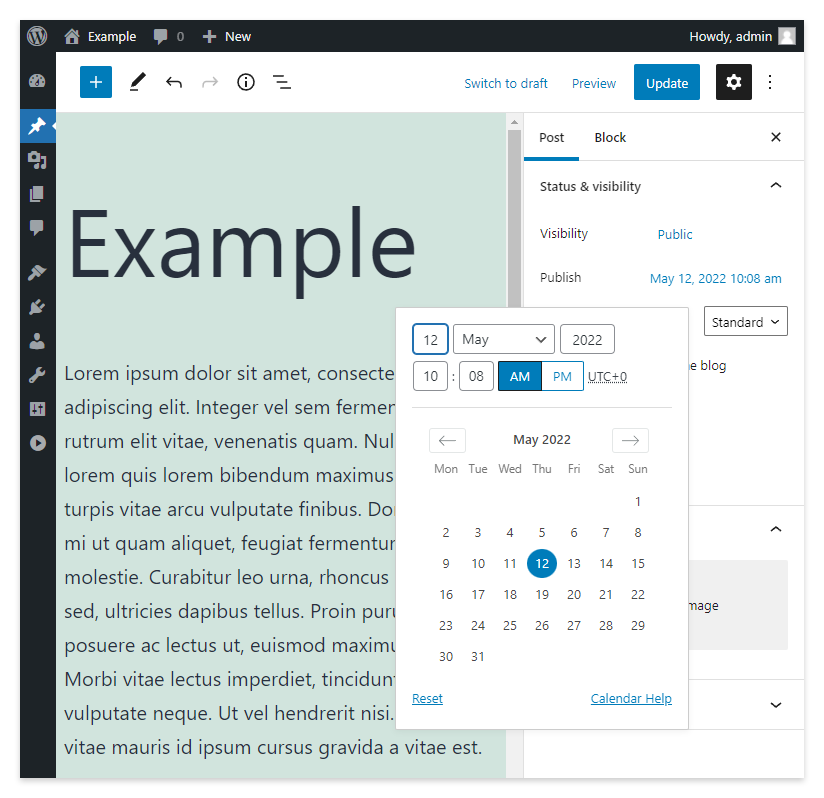
Note that this method is effective only if the number of articles is limited. Moreover, if your theme displays the date of the article, you might also consider removing it from your site.
Display a random post with a plugin
The Random Post on Refresh plugin generates a random post in place of the normal content of a post, page, or custom post type.
To use this plugin, include the plugin shortcode in the content of a post. The plugin will generate a random post from a pool of results defined with the shortcode options.
For instance, to generate a random post taken from the portfolio
custom post type, use the plugin shortcode random-post-on-refresh
and its post_type
parameter.
[random_post_on_refresh post_type="portfolio"]
Note that more parameters are available and documented on the plugin page.
Display related posts in random order
Related posts are a common feature used to automatically display lists of posts at the end of the WordPress article. Many WordPress websites use this solution to keep the users engaged for longer periods of time.
The related posts are usually generated by relevance, for example, by comparing characteristics like the post title, date, or categories of the articles. However, with certain related posts plugins, you can configure a random order.
With one of the plugins I tested, the Contextual Related Posts plugin, you can generate the related posts in random order. To enable this option, proceed to the List Tuning tab of the plugin settings and set “Randomly” with the Order posts selector.
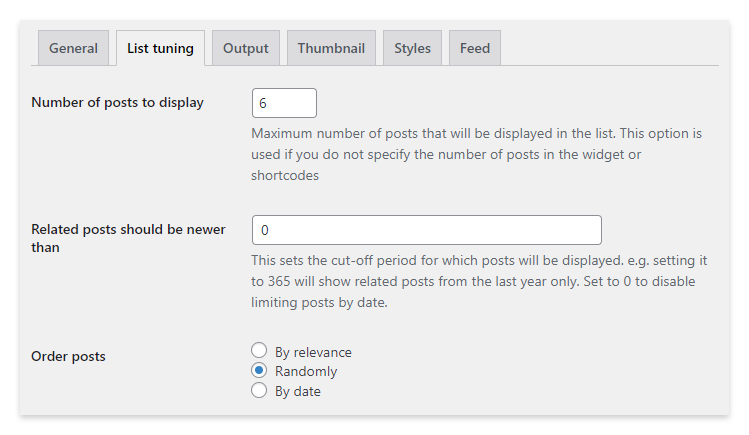
Create a WordPress Gallery in random order
The Random Gallery plugin gives users the ability to generate a gallery of random images.
To use this plugin, add the plugin shortcode in your article and use the shownum
parameter to set the number of images displayed in the gallery and ids
to configure a list of considered images.
The example below will display two random images from a pool of four images, specifically the images with ids 10, 11, 12, and 13.
[random-gallery ids='10,11,12,13' shownum='2']
Note that you can find the ids of the images in the URLs of the Media Library or by inspecting the raw HTML of the article.