If you need to manually implement the Google Ads conversion tracking in a WordPress website that uses WooCommerce, in this article, you can find the code and all the implementation details.
Retrieve the necessary data from your Google Ads account
To retrieve the Conversion Id and the Conversion Label associated with the conversion action, proceed as follows:
- Log in to your Google Ads account
- Visit the Tools & Settings -> Conversions menu
- Select the conversion action that you want to track
- Open the Tag Setup section
- Copy the Conversion Id and the Conversion Label from the provided event snippet
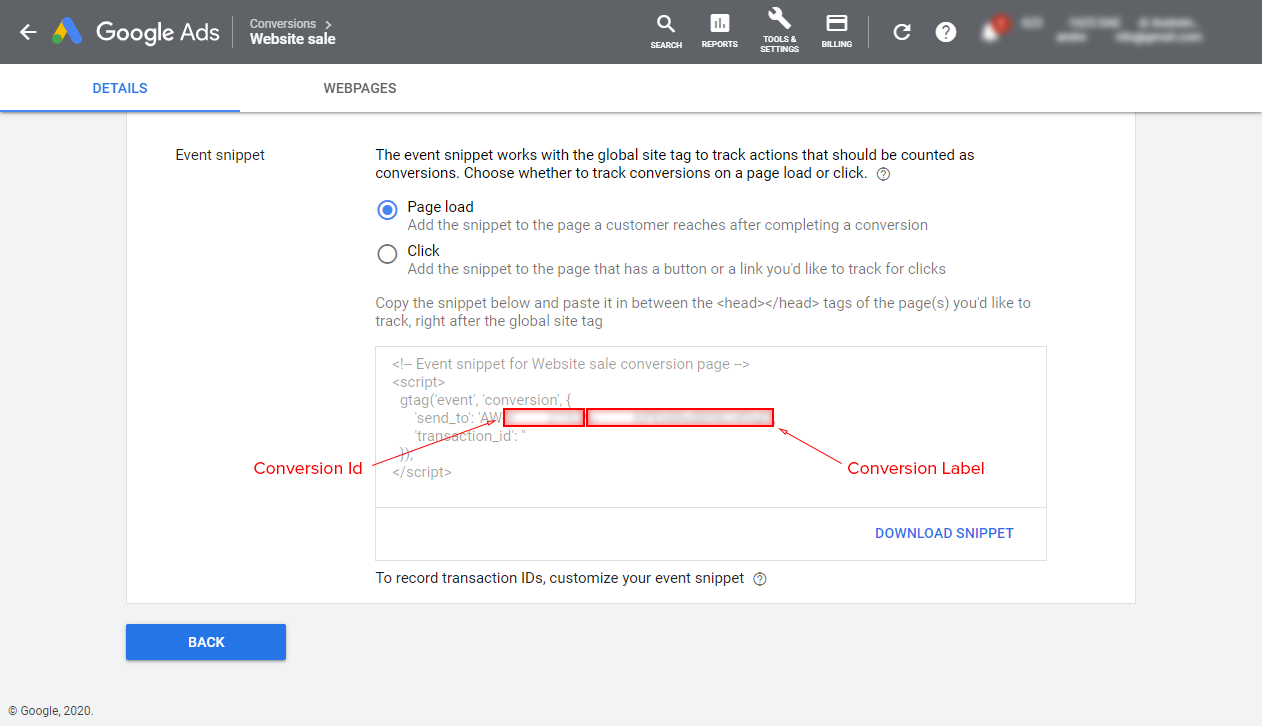
Interact with WordPress
The tracking code should be added to the head section of the page. For this reason, the wp_head hook will be used.
function google_ads_conversion_tracking(){ //Print the tracking code } add_action( 'wp_head', 'google_ads_conversion_tracking' );
Implement the Global Site Tag
The Google Site Tag, available in the Tag Setup section of the conversion action, should be added to all the website pages.
To make the code more reusable, the Conversion Id is first saved in a variable and the added to the snippet properly escaped.
function google_ads_conversion_tracking(){ $conversion_id = '123456789'; ?> <script async src="https://www.googletagmanager.com/gtag/js?id=AW-<?php echo esc_js($conversion_id); ?>"></script> <script> window.dataLayer = window.dataLayer || []; function gtag(){dataLayer.push(arguments);} gtag('js', new Date()); gtag('config', 'AW-<?php echo esc_js($conversion_id); ?>'); </script> <?php } add_action( 'wp_head', 'google_ads_conversion_tracking' );
Implement the Event Snippet
In this section, we will apply the Event Snippet provided by Google Ads on the “Order Received” page. (the page where WooCommerce redirects the user after a purchase)
Detect the order received page
The “Order Received” page can be identified by using the is_order_received_page()
function provided by WooCommerce.
if ( is_order_received_page() ) { //Generate the order object //Retrieve the order number //Verify the order status //Print the event snippet }
Retrieve the order number
The order number can be generated from the order object with the following instructions:
$order_key = $_GET['key']; $order = new WC_Order( wc_get_order_id_by_order_key( $order_key ) ); $order_number = $order->get_order_number();
Alternative methods to achieve this are also possible, like extracting the order number with a regular expression from the $_SERVER
variable.
Verify the status of the order
With the $order
object created in the previous step, you can verify the status of the order. The event snippet will not be generated if an order has the “failed” status.
if ( ! $order->has_status( 'failed' ) ) { //Print the event snippet }
Generate the output
The data required by the event snippet are now available and can be printed in the head section.
<script>
gtag('event', 'conversion', {
'send_to': 'AW-<?php echo esc_js($conversion_id); ?>/<?php echo esc_js($conversion_label); ?>',
'transaction_id': '<?php echo intval($order_number, 10); ?>'
});
</script>
The complete code
Enjoy the complete code. Use it in your functions.php theme file or create a plugin.
function google_ads_conversion_tracking(){ $conversion_id = '991482192'; $conversion_label = 'uBGpCKPEkMwBENCi49gD'; ?> <script async src="https://www.googletagmanager.com/gtag/js?id=AW-<?php echo esc_js($conversion_id); ?>"></script> <script> window.dataLayer = window.dataLayer || []; function gtag(){dataLayer.push(arguments);} gtag('js', new Date()); gtag('config', 'AW-<?php echo esc_js($conversion_id); ?>'); </script> <?php if ( is_order_received_page() ) { $order_key = $_GET['key']; $order = new WC_Order( wc_get_order_id_by_order_key( $order_key ) ); $order_number = $order->get_order_number(); if ( ! $order->has_status( 'failed' ) ) { ?> <script> gtag('event', 'conversion', { 'send_to': 'AW-<?php echo esc_js($conversion_id); ?>/<?php echo esc_js($conversion_label); ?>', 'transaction_id': '<?php echo intval($order_number, 10); ?>' }); </script> <?php } } } add_action( 'wp_head', 'google_ads_conversion_tracking' );
Feel free to expand this code with new functionalities based on your needs, such as the order value or the currency.