This tutorial describes different methods to color specific cells of the tables created with WordPress. Use this information to highlight cells that deserve attention, mark cells that belong to particular categories, or color specific areas to increase the table readability.
Color the individual table cells with custom CSS
Unfortunately, the Table block, the default tool used to include tables in WordPress, doesn’t have an option to customize the color of individual cells. However, we can assign a class that identifies the table with the Additional CSS class(es) field available in the editor sidebar and then use this class in our CSS to color the cells.
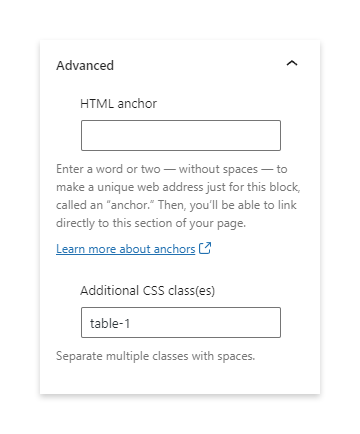
To add the CSS rules, we can either edit the style.css file of the active theme from the Appearance -> Theme File Editor menu or use the theme Custom CSS field when provided by the theme.
In the following example, we will color the table cells of a table identified by the .table-1
selector. We use :nth-child
applied to the tr
and td
elements to target the specific rows. Note that :nth-child
is a CSS pseudo-class that allows us to match siblings based on their position in the group.
It’s worth noting that I also added !important
in the final code. With this directive, the specified table cell will receive our custom color even if there are other competing CSS rules with a higher CSS specificity.
.table-1 > table > tbody > /* Here we target our custom table selector to apply the style changes only to this table. */ tr:nth-child(2) > /* Select the table body row where you want to color the cell, in this case the second row. */ td:nth-child(3) { /* Select the column where you want to color the cell, in this case the third row. */ background: #cccccc !important; }
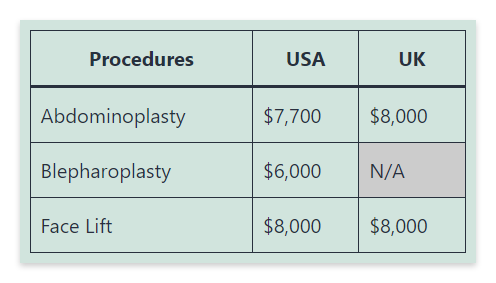
To target cells in the table header, you should slightly change the script above to match the different HTML elements of the table header.
.table-1 > table > thead > tr > /* Select the table header. */ th:nth-child(2) { /* Select the second column of the table header. */ background: #00ff00 !important; }
Note that you can also change the text color of a specific cell with the color
CSS property. This step is sometimes necessary to properly contrast the background color.
/* In this example we made the background color of a table cell dark gray and the text color white to create the appropriate contrast. */ .table-1 > table > tbody > tr:nth-child(3) > td:nth-child(4) { background: #333333 !important; color: #ffffff; }
Color WordPress table cells with table plugins
Plugins are necessary to get advanced table features like sorting, search, data import, etc. Consequently, a large number of users make use of them in their blogs.
Let’s see how to color the table cells with the most common table plugins for WordPress.
TablePress
This section will cover how to perform this process with the TablePress plugin, the most popular table plugin for WordPress.
With the TablePress plugin, there are no dedicated cell coloring features, and the solution still requires the use of custom CSS. The good thing is that TablePress adds classes with names that include the row and column number. Consequently, this CSS implementation is simpler than the one used in the previous tutorial, where we were forced to use pseudo-classes.
/* In this example I colored in blue a cell of a table generated with TablePress */ .tablepress-id-1 /* Target the table with its ID. */ .row-2 /* Target the second row. */ .column-3 /* Target the third column. */ { background-color: #0000ff; color: #ffffff; }
For more information on customizing TablePress with custom CSS, check out the tablepress FAQ, where they explain where to place the CSS code, how to color single rows, how to highlight certain cells, etc.
wpDataTable
Things are much easier with the wpDataTable plugin, where options to color specific cells are available in the UI. More precisely, with this plugin, you can color a cell with the Text Color and the Background Color options available in the toolbar.
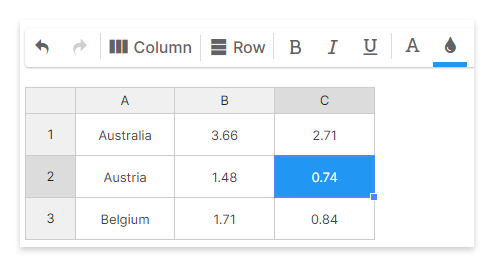
Note that you can also color multiple cells in a single operation if needed. In case, simply select multiple cells as you do on a standard spreadsheet. Then, use the same two UI options again to apply the colors.
League Table
With the League Table plugin, you can modify various aspects of the cells with a cell-specific setting section named Cell Properties. For example, with these settings, you can adjust the cell colors, apply typographic changes, add images and links, customize the cell HTML, and more.
The options to perform this job are the Background Color and the Text Color cell properties.
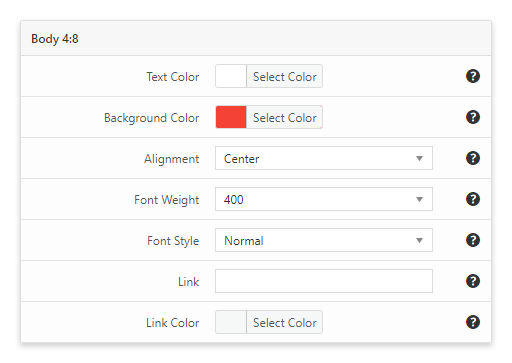
Additional methods to color WordPress table cells
Color table cells with inline styles
While adding inline styles with the block editor is not recommendable. With the Classic Editor, you can edit the post HTML without error messages.
To add inline styles with the Classic Editor, proceed as follows.
- With the Classic Editor active, switch the editing mode from “Visual” to “Text”.
- Write or paste the HTML of a table.
- Add the inline styles.
Below is an example of a table with inline styles used to color a cell:
<table> <tr> <th>Student</th> <th>Pyshics</th> <th>Chemistry</th> </tr> <tr> <td>Sebastian</td> <td style="background: #cccccc;">45</td> <td>75</td> </tr> <tr> <td>Chris</td> <td>62</td> <td>70</td> </tr> </table>
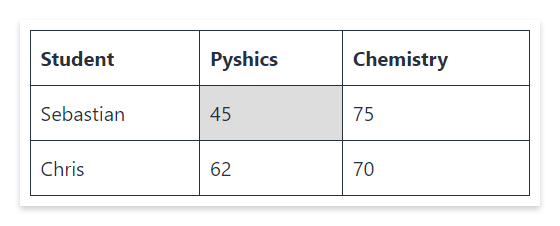
Color table cells with JavaScript
With JavaScript, you can automate the coloring of the table cells based on custom criteria. For example, you can automatically highlight cells with certain numeric values, color rows and columns based on their positions on the table, etc.
For example, the following JavaScript script automatically highlights values higher than 1000.
const table = document.querySelector("table"); for (const row of table.rows) { for (const cell of row.cells) { if(cell.innerText > 1000){ cell.style.backgroundColor = '#ff0000'; } } }